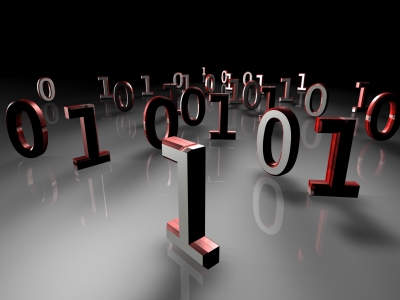
First off, I’d like to say that I’m writing these preliminary posts in a way that I’ll assume you have very little knowledge in programming. I want this content to provide anyone “walking in off the street” the knowledge to be able to write their first program with the Java programming language with as little pain as possible.
So, let’s get started with our first topic: The 5 basic concepts of any programming language. You might say, “Why are we talking about any programming language? I thought this was about Java”. Well, I’ve found that it’s important to remember that a lot of programming languages are very similar, and knowing what’s common between all programming languages will help you transition into any other programming language if you need to! For example, with the Java programming knowledge I had obtained, it took me less than a month to learn how to program in a language called Objective C (which is used for iPhone apps). That’s powerful stuff!
So here are the 5 basic concepts of any programming language:
- Variables
- Control Structures
- Data Structures
- Syntax
- Tools
I recognize that these words probably look foreign to you, but don’t worry, I’ll do my very best at taking the mystery out of them. Now, there’s a lot to say about each of these 5 concepts, so for today’s post I’ll only be talked about item #1, variables!
What is a variable?
Variables are the backbone of any program, and thus the backbone of any programming language. Wiki defines a variable as follows:
In computer programming, a variable is a storage location and an associated symbolic name which contains some known or unknown quantity or information, a value.
Okay, well, that’s kind of cryptic. To me, a variable is simply a way to store some sort of information for later use, and we can retrieve this information by referring to a “word” that will describe this information. For example, let’s say you come to my website www.howtoprogramwithjava.com and the first thing I want to do, is ask you what your name is (so that I can greet you in a nice way the next time you visit my website). I would put a little text box on the screen that asks you what your name is… that text box would represent a variable! Let’s say I called that text box ‘yourName’, that would be the symbolic name (or “word”) for your variable (as described from our wiki definition above). So now, when you type your name into the text box, that information would be stored in a variable called ‘yourName’. I would then be able to come back and say “What value does the variable ‘yourName’ contain?”, and the program would tell me whatever it was your typed into that text box.
This concept is extremely powerful in programming and is used constantly. It is what makes Facebook and Twitter work, it’s what makes paying your bills via your online bank work, it’s what allows you to place a bid on eBay. Variables make the programming world go ’round.
Now, if we want to get more specific, when it comes to the Java programming language, variables have differenttypes. Brace yourself here, as I’m going to try to confuse you by explaining an important concept in three sentences. If I were to be storing your name in a variable, that type would be a String. Or, let’s say I also wanted to store your age, that type would be stored as an Integer. Or let’s say I wanted to store how much money you make in a year, that type would be stored as a Double.
What the heck are String, Integer and Double?
Excellent question! In Java, the programming language wants to know what kind of information you are going to be storing in a variable. This is because Java is a strongly typed language. I could teach you about what the difference is between a strongly typed language and a weakly typed language, but that will likely bore you right now, so let’s just focus on what a type is in Java and why it’s important.
Typing in Java, allows the programming language to know with absolute certainty that the information being stored in a variable will be ‘a certain way’. So like I said, if you’re storing your age, you would use the Integer type… well that’s because in Java, an Integer means you have a number that won’t have any decimal places in it. It will be a whole number, like 5, or 20, or 60, or -60, or 4000, or -16000. All of those numbers would be considered anInteger in Java. So what would happen if you tried to store something that wasn’t an Integer, into an Integer variable, say for instance the value “$35.38″? Well, quite simply, you would get an error in the program and you would have to fix it! ”$35.38″ has a dollar sign ($) in it, as well as a decimal place with two digits of accuracy. In Java, when you specify that a variable is of type Integer, you are simply not allowed to store anything except a whole number.
Now I don’t want to go into too much detail about Types, as this is better suited to programming basic concept #3 – Data Structures. So that’s all I will touch on for now, but no worries, it will all make sense in time!
So, to sum up, we talked about what a variable is and how you can store information in a variable and thenretrieve that information at some later point in time. The variable can have a name, and this name you give to the variable is usually named after the kind of content you’ll be storing in the variable, so if I’m storing your name in the variable, you’d name the variable ‘yourName’. You wouldn’t HAVE to give it that name, you could name the variable “holyCrapImProgramming”, but that wouldn’t make a whole lot of sense considering you are trying to store a person’s name. Makes sense right? Finally, variables have types, and these types are used to help us organize what can and cannot be stored in the variable. Hint: having a type will help to open up what kind of things we can do with the information inside the variable. Example: if you have two Integers (let’s say 50 and 32), you would be able to subtract one variable from the other (i.e 50 – 32 = 18), pretty straight forward right? But, if you had two variables that stored names (i.e. “Trevor” and “Geoff”) it wouldn’t make sense to subtract one from the other (i.e. ”Trevor” – “Geoff”), because that just doesn’t mean anything! So, types are also a powerful thing, and they help us to make sense of what we CAN do with our variables and what we CANNOT do!
So I hope this information has been helpful to you, and I hope you realize what the benefits of learning a programming language are! The allure to learning a programming language is quite high in today’s corporate world, as most companies are hiring programmers with the skills to create web applications. The programming profession is one that provides excellent pay and job stability, and in the end, isn’t that what we’re all looking for?
We’ve already discussed what a variable is, so now let’s talk about control structures. What on earth is a control structure!? Wiki describes it as follows:
A control structure is a block of programming that analyzes variables and chooses a direction in which to go based on given parameters. The term flow control details the direction the program takes (which way program control “flows”). Hence it is the basic decision-making process in computing; flow controldetermines how a computer will respond when given certain conditions and parameters.
H’okay, so, that definition is obviously a bunch of technical terms that no beginner to programming would understand. So let me try to describe it in more human terms. When a program is running, the code is being read by the computer line by line (from top to bottom, and for the most part left to right), just like you would read a book. This is known as the “code flow“, now as the code is being read from top to bottom, it make hit a point where it needs to make a decision, this decision could make the code jump to a completely different part of the program, or it could make it re-run a certain piece again, or just plain skip a bunch of code. You could think of this process like if you were to read a choose your own adventure book, you get to page 4 of the book, and it says “if you want to do X, turn to page 14, if you want to do Y, turn to page 5″. That decision that must be made by the reader is the samedecision that the computer program must make, only the computer program has a strict set of rules to decide which direction to go (whereas if you were reading a book, it would be a subjective choice based on whomever is reading the book). So, this decision that must be made, that will in turn effect the flow of code, is known as a control structure!
Okay, that doesn’t seem to be such a hard concept… a control structure is just a decision that the computer makes. So then that begs the question, what is it using to base that decision on? Well, it’s simply basing its decision on the variables that you give it! Let me show you a simple example, here’s a piece of Java code:
if (yourAge < 20 && yourAge > 12) { // you are a teenager } else { // you are NOT a teenager } |
So, you can see above that we have a variable, and its name is
yourAge
, and we are comparing yourAge
to 20 and 12, if you’re less than 20 AND you’re more than 12, then you must be a teenager (because you are between 13 and 19 years of age). What will happen inside of this control structure, is that if the value assigned to the yourAge
variable is between 13 and 19, then the code will do whatever is inside of the first segment (between those first two curly braces { } ), and it will skip whatever is inside of the second code segment (the second set of curly braces { } ). And if you are NOT a teenager, then it will skip the first segment of code and it will execute whatever is inside of the second segment of code.
Let’s not worry too much about what the code looks like for the moment, as I’ll touch on how to write the code out properly in section #4 syntax. The only concept you need to try and wrap your head around right now, is that there is a way in programming to ‘choose’ which lines of code to execute, and which lines of code to skip, and that will alldepend on the state of the variables inside of your control structure. When I say state of a variable, I just mean what value that variable has at any given moment, so if
yourAge = 15
, then the state of that variable is currently 15 (and thus, you’re a teenager).
You’ve now seen one control structure and I’ve tried to explain it as best I could. This control structure is known as an
if...else
structure. This is a very common control structure in programming, let me hit you with some other examples. Here’s a while loop
control structure:while (yourAge < 18) { // you are not an adult // so keep on growing up! } |
This
while loop
control structure is also very handy, its purpose is to execute code between those curly braces { }over and over and over until the condition becomes false. Okay, so what’s the condition? Well, the condition is between the round brackets ( ), and in this example it checks yourAge
to see if you are less than 18. So if you are less than 18, it will continuously execute the code inside the curly braces { }. Now, if you were 18 or older before thewhile loop
control structure is reached by the code flow, then it won’t execute ANY of the code inside of the curly braces { }. It will just skip that code and continue on executing code below the while loop
control structure.
There are a few other examples of control structures in Java, but I don’t want to overwhelm you with them right now. So instead I’ll sum up what we’ve learned today.
We’ve learned that code flows from top to bottom and for the most part left to right, just like a book. We’ve learned that we can skip over certain code or execute certain parts of code over and over again, and this is all achieved by using different control structures. These control structures are immensely important to programming, as they make the programs function properly. For example, you wouldn’t want people to be able to login to your Facebook account if they enter the wrong password right? Well, that’s why we use the
if...else
control structure, if the passwords match, then login, else show a “password is incorrect” screen. So, without control structures, your program’s code would only flow in one way, and it would essentially only do one thing over and over again, which wouldn’t be very helpful. Changing what the code does based on a variable is what makes programs useful to us all!
Data structures, what are they, why are they useful? Well, let’s turn to a quick definition from wiki:
In computer science, a data structure is a particular way of storing and organizing data in a computer so that it can be used efficiently.
Okay, that definition is a little more down to earth. But, there is a lot of ‘meat’ behind data structures. Let me try to explain the point of data structures by giving you an example… Let’s use a list of contacts as the example! You probably have a list of contacts somewhere in your life, whether it’s in one of your email programs or an address book in a kitchen drawer. There are a bunch of contacts, and that list of contacts could grow (or shrink) at any given moment. If you were to try and represent all those contacts as variables in a computer program, how would you do it? Well, there’s a right way, and a wrong way. For the purposes of our example, let’s say we need to keep track of 10 contacts.
First, the WRONG WAY:
If we need to store 10 contacts, we would probably define 10 variables, right?
String contact1, contact2, contact3, contact4, contact5, contact6, contact7, contact8, contact9, contact10; contact1 = "John Smith (john.smith@someCompany.com)"; contact2 = "Jane Smith (jane.smith@someCompany.com)"; contact3 = //... etc. |
In the world of programming, this is just a terrible way of trying to store 10 different variables. This is because of two main reasons:
- The sheer amount of text that you’ll need to write in your program
- Sure, right now we only have 10 contacts, so it’s not too bad, but what if we had 1,000 contacts! Imagine typing that out a thousand times! Forget about it!
- The flexibility of code
- If we need to add another contact, we wouldn’t be able to do it without manually editing our code. We would have to go into our code, physically write out
contact11
, and then try to store whatever information is needed into the new variable. This is just crazy talk!
- If we need to add another contact, we wouldn’t be able to do it without manually editing our code. We would have to go into our code, physically write out
So, what is the RIGHT WAY?
I’m glad you asked, it’s a data structure of course!
In this case, we have a list of contacts, and with Java there is a data structure called a List! Okay, so what does it look like? Here’s the code:
List contacts = new ArrayList(); |
Again, let’s not worry about all those symbols and confusing brackets and semi-colon, we’ll cover that later. All I need you to understand right now, is that there is a way to store a bunch of contacts into something called a data structure (in the case of our example, a
List
). Okay, so, what’s so great about a List? Well, for one thing, you can add and remove things from a list with ease. So if you started with 10 contacts, it’s a piece of cake to add another contact to the list, how you ask? Just like this:contacts.add("John Smith (john.smith@someCompany.com)"); contacts.add("Jane Smith (jane.smith@someCompany.com)"); |
Voila, we’ve added another contact to our contacts list! So, you may be saying, the right way looks like just as much typing as the wrong way. You’ve got a point, but the main difference is that with the first approach, you had to “create” 10 unique variables (i.e.
contact1, contact2, contact3, contact4
…), but with the second approach, we only created 1 variable (contacts
). Because we only had to create 1 variable, and because we can saycontacts.add(someRandomContact)
, means that our code is much more flexible and dynamic. When I saydynamic, I mean that the outcomes of the program can change depending on what variables you give to it. That’s really the key to using data structures! We want our code to be as dynamic as possible, we want it to be able to handle a bunch of situations without having to write more and more code as the days go by.
In essence, a data structure is just a way to get around having to create tons of variables. Now, there are a bunch of other data structures in Java, but I’ll just quickly touch on the ones we use most often when programming. The next one I’ll talk about is the
“Honda” -> “Civic”, “Prelude”
“Toyota” -> “Corolla”, “Celica”, “Rav4″
“Ford” -> “Focus”, “Mustang”
“Audi” -> “R8″
HashMap
. This doesn’t sound as straightforward as our last data structure (the List), but it’s really just a fancy name for a fairly simple concept. So, what is a hash map? Here’s a visual representation:
What you see here, is the make of a car on the left, which points to different models of that make. This is known in the programming world as a Key/Value pair. The key in this case, is the Make of the car (Toyota, Honda, Ford, Audi), the value in the case, is the model (Civic, Corolla, Focus, R8). That is how a
HashMap
works, and it’s just another data structure! This concept is use all over the place on the web. For example, if you have ever filled out a quote for automobile insurance online, you’ve probably had to choose the make and model of your car. I bet you that information was presented on screen by using a HashMap
(just like I showed you above)! Because when you select “Toyota” from one drop down list, the second drop down list will need to change its contents to only show models of cars made by Toyota. Then if you change the first drop down to Honda, that second drop down list will change again to show cars made by Honda.
So how about I show you what a
HashMap
looks like in Java code?Map |
Wow, there’s a lot of stuff going on in there, but don’t be intimidated, all you need to understand right now is the concept that this
HashMap
data structure stores key/value pairs. In our case, a make is the key, and a List
of models is our value. Now, that’s kind of interesting, did you see what we did there? I just said that we have a HashMap
data structure, and inside the HashMap
we are storing a List
(which is another data structure). This is done on a fairly regular basis in the programming world. We can use one data structure inside of another! So, if the point of data structures is to help us minimize the number of variables we need to create, then we are really saving ourselves the hassle of creating a lot of variables!
Okay, so let’s sum up everything we’ve talked about. A data structure is a way of storing and organizing data in such a way that it can be used efficiently. The point is to avoid creating crap loads of variables people! Java allows us to do this by using
Lists
and HashMaps
(as well as many other data structures, but these two are the most common). These two data structures can grow and shrink without you having to worry about coding all of that ‘behind the scenes’ stuff. When you add to a List
, the new element is there and is usable (like magic), when you remove from theList
, that element is now gone (poof!). This saves us from creating and deleting variables in our code manually.
Alright, that about sums up what I need you to know for our next section: syntax. In my opinion as a veteran programmer, syntax is the one thing that will discourage you from learning to program. It takes practice and patience to understand, but I promise you, it will become second nature in time. So please be patient, and more importantly, be excited to learn about syntax in Java, because once you get the hang of it, you’ll be on your way to programming like a champ!
What is syntax? As always, let’s hop over to wiki for a quick definition:
In computer science, the syntax of a programming language is the set of rules that define the combinations of symbols that are considered to be correctly structured programs in that language.
Alright, so I would say that’s almost English, but what do they mean by “combinations of symbols that are correctly structured”? Well, I would choose a different word than symbols. I would define syntax to be a particular layout ofwords and symbols. An example of this in the case of Java would be round brackets (), curly brackets {}, and variables, among other things. Think of it like this, when you look at an email address (i.e.john.smith@company.com), you can immediately identify the fact that it’s an email address right? Sowhy is that? Why does your brain make the connection that it’s an email address, and not, say, a website address? Well, it’s because an email address has a particular syntax. You need some combination of letters and numbers, potentially with underscores (_) or periods (.) in between, followed by an at (@) symbol, followed by awebsite domain (company.com) That is a defined combination of letters and symbols that are considered correct structure in the “language” of the internet and email addresses. So, syntax in a programming language is much the same, there are a set of rules that are in place, which when you follow them, allows your programminglanguage to understand you and allow you to create some piece of functioning software. But, if you don’t abide by the rules of a programming languages’ syntax, you’ll get errors 

How about an example of syntax in Java? Well you’ve seen it already back when we talked about variables and control structures. To define a variable in Java, you need to do this:
String helloVariable = "Hello Everyone!"; |
There are four parts to the syntax of creating a variable in Java. The first is the word
String
, this is the variable’stype. Remember when we talked about variable types in the first part of this series? I mentioned String
, Integer
and Double
, three different variable types that allow you to store three different kinds of data. A String
in this case, allows you to store regular letters and special characters. The second part to this variable creation syntax is the variable name, in this case I arbitrarily chose helloVariable
. I could have just as easily chosenholyCowThisIsAVariableName
. Variable names can be made up of letters and numbers, but the only special characters they can contain are underscores (_). They also usually start with a lower case letter, they don’t have to, but that’s kind of an accepted and suggested convention (at least in the Java world). The third part of the syntax for creating a variable is the value that the variable will hold. In this case, we have a String
variable, so we have the value"Hello Everyone!"
. In java, Strings are defined by wrapping regular letters/numbers/special characters in quotes (” “). Again, that’s just the syntax that Java uses. The last part of this syntax, is the part that marks this particular code segment as being complete. In Java, we use the semi-colon (;) to mark a part of our code as complete. You will see that almost every line of code in Java will end with a semi-colon (;). There are certain exceptions to this, for example control structures aren’t marked with semi-colons, as they use curly braces to make their beginning and end. Think of it like putting a period at the end of every sentence. If we didn’t put a period, we would just have one long unstructured run on sentence, and that wouldn’t help us to understand anything that’s being said.
So, as I mentioned before, the syntax of any programming language will likely be your biggest hurdle as a new developer, but as you see more and more examples of code and are introduced to more and more syntax in the language, you will become comfortable. There is good news though, as people have realized that dealing with syntax can be tough, so certain companies (or groups of enthusiasts, a.k.a nerds) have created tools to help us with the syntax of programming languages. These tools are called IDEs, or, Integrated Development Environments, which you can download onto your computer and use to create programs. These IDEs have built in syntax checkers (much like the grammar checker in MS Word) that will let you know if your syntax is incorrect, and will even give you hints with what it thinks you meant to put! So don’t you worry, I’ll cover those tools in the next section of this 5 part series.
So, let’s sum up. In today’s post, we have learned that syntax just means that there’s a “correct” way to write down your code, and that this allows the programming language understand what it is that you’re trying to tell it to do. Unfortunately for us, computer’s can’t read our minds (yet!) and know what it is that we want them to do, so some very smart people have created this “computer language” that, when understood by programmers, allows us to tell the computer what actions we would like it to carry out… whether that action be to send a bill payment to our credit card company, or to play a game of poker online with a virtual table full of strangers. Syntax is our systematic way to talk to a computer and convey our wishes.
I hope I have taken a little bit of mystery out of the term syntax, and I look forward to teaching you about our final subject… tools! A developer’s best friend 

What are tools? Well, I don’t think we need to go to wiki to define this one, as many of you should already know what a tool is. In the real world, a tool is something (usually a physical object) that allows you to get a certain job done in a more timely manner. Well, this holds true with the programming world too. A tool in programming is a piece of software that, when used while you code, allows you to get your program done faster!
There are probably tens of thousands, if not hundreds of thousands of different tools across all the programming languages, but I’ll focus on the main kinds tools that everyone is likely to use.
The first and most important tool, in my opinion, is an IDE. An Integrated Development Environment is a piece of software that will make your coding life so much easier. IDEs will check the syntax of your code to ensure you don’t have any errors, they will organize your files and give you a nice way to view them (i.e. applies colour schemes to your code so it’s easier to interpret), they tend to have code completion (which will actually fill in some code for you, in common scenarios), as well as allow you to navigate through your code easily. There are many other advantages of using an IDE, but I think you get the idea. In the Java world, the IDE I use most often is:
- Spring (STS)
This IDE is free and full of features, it’s what I use whenever I program (both at work and at home)
For the purposes of this java tutorial, I will focus on just this one tool, as it will be the most important thing you use when you begin programming. So, let’s install Spring STS! The first thing you’ll need to know is that the Spring STS IDE, and all IDEs in Java, require the Java Development Kit to be installed on your computer, so how about wedownload that now as well here.
Step 2 – Run the downloaded file and leave all options default as you click next
Special Note: This installer will likely popup another installer for the Java Runtime Environment (JRE), so if it looks like your install has stopped for no reason, check to see if you have any other pop-ups that may be hidden that require you to click Next.
Step 3 – Click this link to go to the Spring STS download page and skip the registration (if you like):
Step 4 – Choose the appropriate version of STS to install, I’ve highlighted the windows versions but I believe there is a MAC version as well:
Step 5 – Now, once you’ve downloaded that file, run it and start the installation process. Leave all the options set to their defaults as you click next, until you get to this screen.
Here you will choose the directory where you installed your Java SDK, the default location is: ‘C:\Program Files\Java\jdk1.6.0_33′
There, now you have successfully installed Spring STS and you are ready to begin programming! That’s all I will cover on tools for today, as I will leave the discussion of other tools to future posts as they are required. So, thank you for joining me on this five part series about the basic concepts of the programming languages, you are now ready to learn how to write your first java program (also known as the Java Hello World program). If you have any questions about this series, by all means leave a comment and I will get back to you as quickly as I can!
Regards
Owntutorials | Cameron Thomas
MaximeTech | Best IT solutions Provider | Web Development |Mobile Applications
No comments:
Post a Comment